Delete All Files Older than x Days using PowerShell
How to Delete Older Files Using PowerShell?
Deleting unnecessary files is important for keeping your computer and storage running smoothly. But manually deleting them can take ages if you have a lot of files to go through.
Fortunately, the Windows PowerShell scripting language provides an easy and efficient way to delete all files older than a certain number of days. In this blog post, we’ll explain how to use PowerShell to delete all files older than x days. Let’s get started!
PowerShell Script to Delete Older Files
To begin, you’ll need to create a Windows PowerShell script. This script will contain the commands needed to delete all files older than x days from a specific directory. To delete all files that are older than a certain number of days in PowerShell, you can use the Get-ChildItem
cmdlet to retrieve the files, and the Where-Object
cmdlet to filter the files based on their age. You can then use the Remove-Item
cmdlet to delete the filtered files.
Here’s an example of how you can do this:
#Parameters $Path = "C:\Temp" # Path where the file is located $Days = "30" # Number of days before current date #Calculate Cutoff date $CutoffDate = (Get-Date).AddDays(-$Days) #Get All Files modified more than the last 30 days Get-ChildItem -Path $Path -Recurse -File | Where-Object { $_.LastWriteTime -lt $CutoffDate } | Remove-Item –Force -Verbose |
This will delete all files in the C:\Temp
directory and its subdirectories that have the last write time that is older than 30 days.
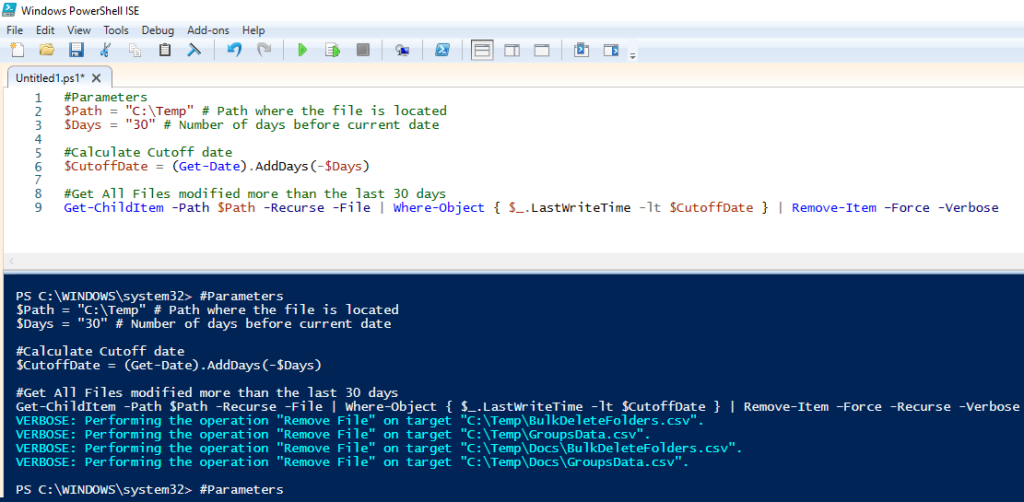
The first line of code sets the path for where the files are located (e.g., C:\Temp).
The second line sets the number of days before today’s date (e.g., 30). You can adjust the value of the $days
variable to specify the number of days that should be used as the cutoff. You can also specify a different folder path by modifying the C:\folder
path.
The third command subtracts the number of days set in the $Days from today’s date and stores and stores it in a variable called “$CutoffDate”.
The fourth command looks through all folders within that path for any file that was last modified before “$CutoffDate” (i.e., more than 30 days ago); if it finds such a file, it deletes it using the “Remove-Item” command. We have used the -Force
parameter to suppress this prompt and delete the files without any confirmation.
What if you want to consider only the Day Instead of the Time Part? Here’s a PowerShell script that deletes log files older than 30 days, considering only the date and not the time:
# Define the path to the log files $LogPath = "C:\Logs" # Get the current date $currentDate = Get-Date # Get all log files in the specified directory $logFiles = Get-ChildItem -Path $logPath -Filter *.log foreach ($file in $logFiles) { # Get the last write date of the file $fileDate = $file.LastWriteTime.Date # Calculate the age of the file $fileAge = (New-TimeSpan -Start $fileDate -End $currentDate).Days # Check if the file is older than 30 days if ($fileAge -gt 30) { try { # Delete the file Remove-Item -Path $file.FullName -Force Write-Output "Deleted $($file.FullName) with age '$fileAge' days" } catch { Write-Output "Failed to delete $($file.FullName): $_" } } } |
Delete Old Files based on the Creation Date using PowerShell
How about deleting old files based on their creation date? For example, instead of the last modified date – Let’s use the “Creation Date” as deletion criteria:
Get-ChildItem C:\Temp -Recurse -File | Where {$_.CreationTime -lt (Get-Date).AddDays(-30)} | Remove-Item -Force |
This will delete all files created more than 30 days ago without prompting for confirmation.
Once you’ve created your script, save it, something like “DeleteOldFiles.ps1” for reference purposes later on. You should now have everything ready to run your script now! You can run it by opening up Windows PowerShell and entering the following command into it: ./DeleteOldFiles.ps1
.
This will execute your script and delete all files in that folder that are older than 30 days old based on their “last write time” attribute (i.e. when they were last modified) or “created time”.
If you want to change how many days old a file needs to be before it gets deleted, simply go back into PowerShell ISE, Notepad, or your text editor of choice and modify the variables to whatever value you wish (e.g., 10). Once done, rerun your script again with ./DeleteOldFiles.ps1
EXAMPLE CODE DELETE FILE OLD 5 DAY WITH FILE LOG AND MULTI FOLDER
Start-Transcript -Path “D:\LOG\log_$(Get-Date -f yyyy-MM-dd_HH-mm-ss).txt” -Force -Append Get-Date -Format “yyyy-mm-dd HH:MM” Try { # Start Try $Path = “D:\FOLDER1″,”D:\FOLDER2” $Date = (Get-Date).AddDays(-5) $TestPath = Test-Path -Path $Path -PathType Container If ( $TestPath -Eq $Null ) { # Start If Write-Host "The $TestPath String is empty, Path is not a valid" } # End If Else { # Start Else Write-host "Path is OK and Cleanup is now running... 0%" $GetFiles = Get-ChildItem -Path $Path $Path2 -Recurse -Force | Where-Object { $_.LastWriteTime -lt $Date } | Remove-Item -Recurse -force -Verbose | Write-host "Path is OK and Cleanup is now running... 100%" -ForegroundColor Green } # End Else } # End Try Catch { # Start Catch Write-Warning -Message “## ERROR## “ Write-Warning -Message “## Script could not start ## “ Write-Warning $Error[0] } # End Catch |
obviously like your website but you need to test the spelling on quite a few of your posts Several of them are rife with spelling problems and I to find it very troublesome to inform the reality on the other hand Ill certainly come back again
Your blog is a constant source of inspiration for me. Your passion for your subject matter shines through in every post, and it’s clear that you genuinely care about making a positive impact on your readers.